eZ Components - ImageAnalysis
Table of Contents
Introduction
The ImageAnalysis component allows you to analyze certain image attributes.
Class overview
ezcImageAnalyzer is the main class for this component. It is responsible for handling the analysis of image files, as well as caching the results.
Usage
MIME type determination
The following example simply detects the MIME type of an image and prints it:
- <?php
- require_once 'tutorial_autoload.php';
- $tutorialPath = dirname( __FILE__ );
- $image = new ezcImageAnalyzer( "{$tutorialPath}/img/imageanalysis_example_01.jpg" );
- echo "Image has MIME type <{$image->mime}>.\n";
- ?>
On line 5, a new ezcImageAnalyzer object is instantiated. This must be done for each image to be analyzed. In line 7, the MIME type is determined. Here is an example image including the output:
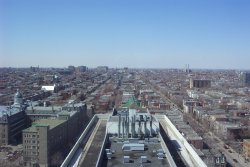
Image has MIME type <image/jpeg>
Extracting further data
Aside from the MIME type, ImageAnalysis can extract other image information. The following example demonstrates this:
- <?php
- require_once 'tutorial_autoload.php';
- $tutorialPath = dirname( __FILE__ );
- $image = new ezcImageAnalyzer( $tutorialPath.'/img/imageanalysis_example_02.jpg' );
- echo "Image data:\n";
- echo "MIME type:\t{$image->mime}\n";
- echo "Width:\t\t{$image->data->width} px\n";
- echo "Height:\t\t{$image->data->height} px\n";
- echo "Filesize:\t{$image->data->size} b\n";
- $comment = ( $image->data->comment == '' ) ? 'n/a' : $image->data->comment;
- echo "Comment:\t{$comment}\n";
- ?>
The example is basically the same as the first one, except that more data is requested from ezcImageAnalyzer (lines 8 to 11). The analysis of additional data begins on line 9. After that, the data is cached in the ezcImageAnalyzer object.
The width, height and size values are available for every analyzable image. A comment is not always available. If an image property is not available, the output will be some sensible default value (such as n/a). (Note that the availability of some data also depends on the availability of PHP's Exif extension.)
The example image and printed output is shown below:
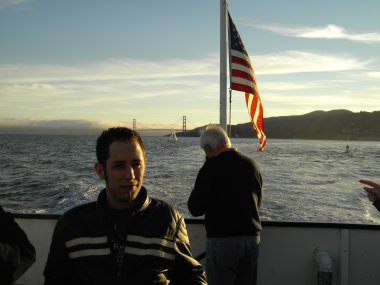
Image data:
MIME type: image/jpeg
Width: 380 px
Height: 285 px
Filesize: 25984 b
Comment: n/a
Configuring handlers
Like ezcImageConverter, ezcImageAnalyzer is based on handler classes, which allow it to utilize different back-ends for image analysis. The currently implemented handlers are:
- ezcImageAnalyzerPhpHandler
- This uses PHP's getimagesize() function (which does not require the GD extension!) and can optionally use PHP's Exif extension.
- ezcImageAnalyzerImagemagickHandler
- Here ImageMagick's "identify" program is used.
Both handlers are activated by default and are capable of determining if their preconditions are fulfilled.
You might need to configure a handler, if for example the path to the ImageMagick "identify" binary is not available in the $PATH environment variable. The following example shows how this is possible and what else can be configured for the handlers:
- <?php
- require_once 'tutorial_autoload.php';
- $tutorialPath = dirname( __FILE__ );
- ezcImageAnalyzer::setHandlerClasses(
- array(
- 'ezcImageAnalyzerImagemagickHandler' => array( 'binary' => '/usr/bin/identify' ),
- )
- );
- $image = new ezcImageAnalyzer( $tutorialPath.'/img/imageanalysis_example_03.jpg' );
- echo "Image data:\n";
- echo "MIME type:\t{$image->mime}\n";
- echo "Width:\t\t{$image->data->width} px\n";
- echo "Height:\t\t{$image->data->height} px\n";
- echo "Filesize:\t{$image->data->size} b\n";
- $comment = ( $image->data->comment == '' ) ? 'n/a' : $image->data->comment;
- echo "Comment:\t{$comment}\n";
- ?>
Basically, the code is the same as in example 2, except that ezcImageAnalyzer is being configured to only use its ImageMagick handler and not the PHP handler. In addition, the location of the "identify" binary is explicitly set. See the results below:
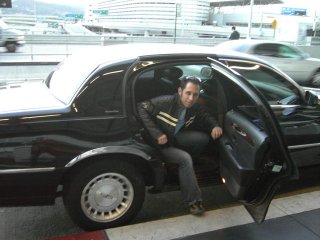
Image data:
MIME type: image/jpeg
Width: 320 px
Height: 240 px
Filesize: 26365 b
Comment: San Francisco airport, October 2005.
More information
For more information, see the ezcImageAnalyzer API documentation.